CRUD API with Next.js and Prisma
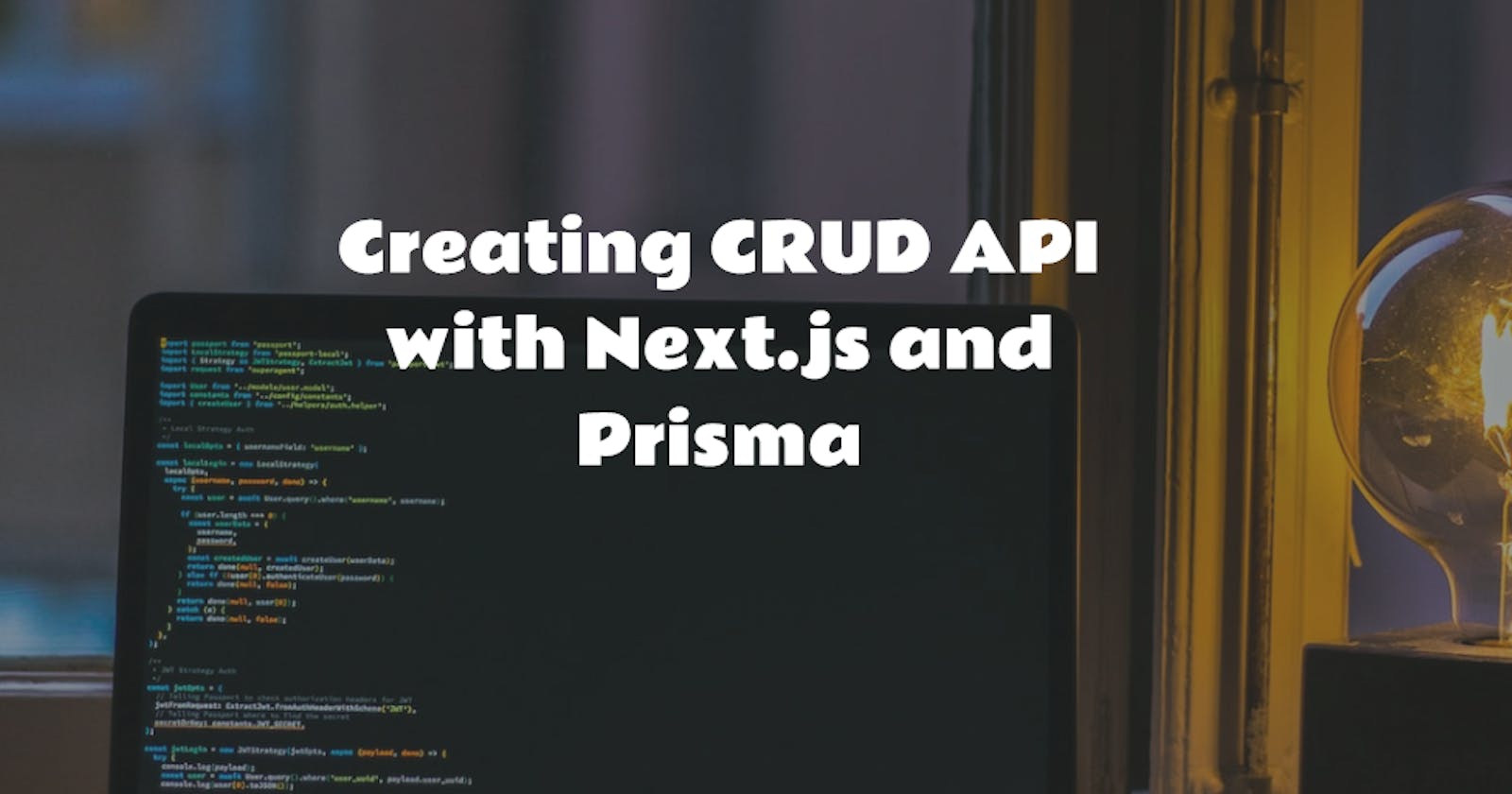
Introduction
I'm currently working on a chat web application, and I'm using Next.js with Prisma for it. In this post, I will show you how I made CRUD API with Next.js and Prisma.
Code
schema.prisma
generator client {
provider = "prisma-client-js"
}
datasource db {
provider = "postgresql" // Use Postgresql DB
url = env("DATABASE_URL") // Get DATABASE_URL from .env.local
}
// Define User model for CRUD API
model User {
id Int @id @default(autoincrement())
username String
}
// Define Message model for CRUD API
model Message {
id Int @id @default(autoincrement())
message String
username String
}
lib/prisma.js
import { PrismaClient } from "@prisma/client";
// Create and export prisma client
const prisma = new PrismaClient();
export default prisma;
users/route.ts
import { NextResponse } from 'next/server';
import prisma from '../../../lib/prisma';
// Get all the user and return as json response
export async function GET() {
const users = await prisma.user.findMany()
return NextResponse.json(users)
}
// Get body and create new user
export async function POST(request: Request) {
const body = await request.json()
const user = await prisma.user.create({ data: body })
return NextResponse.json(user)
}
// Get body and change data on specific Id
export async function PUT(request: Request) {
const { id, username } = await request.json()
const user = await prisma.user.update({
where: { id },
data: { username },
})
return NextResponse.json(user)
}
GitHub: https://github.com/dongjin2008/whisperchat
Conclusion
Hope this information is helpful 😊.
See you on the next post 👋